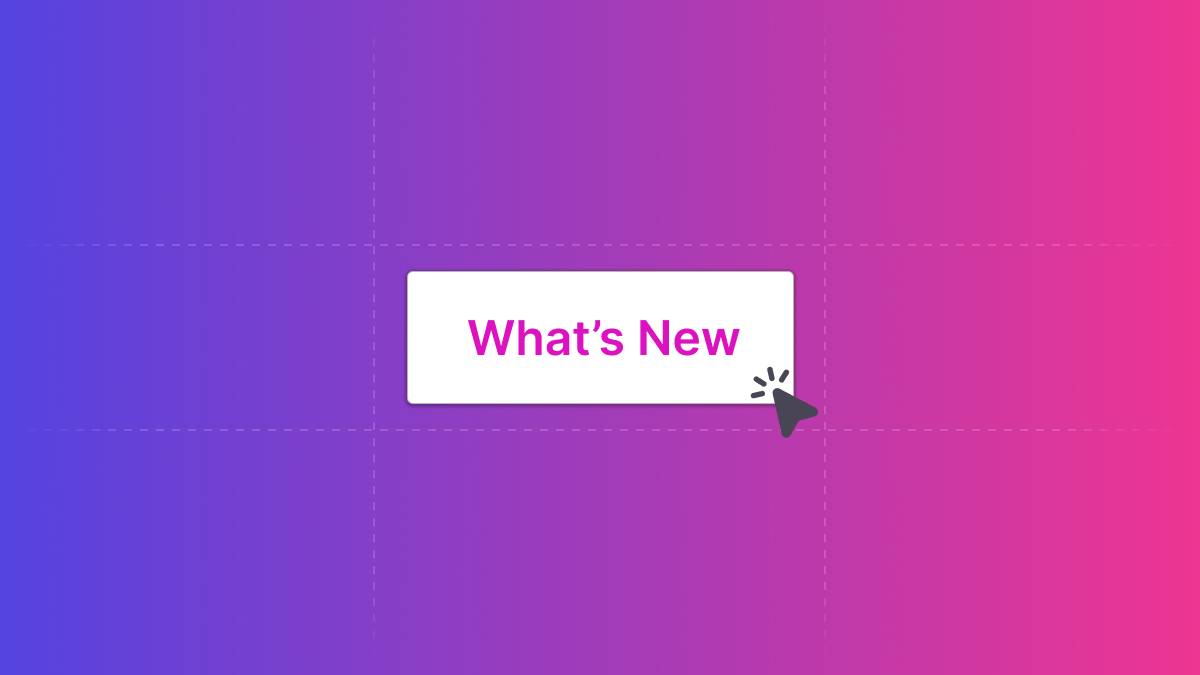
Mar 7, 2023 • TUTORIAL
Create a Custom Widget Launcher
If you want the Widget to open from a specific button, link, or element on your app or website, you can create a custom launcher. For instance, you could set up the Widget to run each time a user clicks the "Changelog" link on your website. This guide will show you how to create a custom launcher that perfectly matches your website.
It is advised to have some basic knowledge of HTML and JavaScript, though it is optional to complete this quickstart. HTML Introduction and JavaScript MDN both have more details.
Adding the Widget to your web page
The Widget can be added to your website in two different ways. The recommended approach is the first method, using the embed script tag. This method involves copying a code snippet from your Onset Dashboard and inserting it into the HTML code of your website. When the page loads, the Widget will be displayed within an iframe on your website.
<script>
window.onsetWidgetSettings = {
triggerText: '🔔 What\'s New',
page: 'PAGE_HOST'
};
(function (e, t) {
e.onsetWidget = {};
e.onsetWidget.on = function () {
(e.onsetWidget.$ = e.onsetWidget.$ || []).push(arguments);
};
var c = t.getElementsByTagName('script')[0],
i = t.createElement('script');
i.async = true;
i.src = 'https://widget.onset.io/widget.js';
c.parentNode.insertBefore(i, c);
})(window, document);
</script>
The second method of embedding the Widget is by installing it via NPM. The process involves downloading the widget package from the NPM repository and integrating it into your website. This method is suitable for developers who want to customize the Widget and have more control over its implementation.
npm install --save @onsetio/widget
yarn add @onsetio/widget
Both methods can achieve the same result of having the Widget displayed on your website, but the embed script tag method is the more straightforward option for most users. The NPM installation method is better suited for more advanced users and web development projects that require more customization and control over the Widget.
Disabling the default launcher
Before using a custom launcher, it is a good idea to disable the Widget's built-in launcher button. To accomplish this, set the customTrigger property to true. This instructs the Widget that you prefer to use a different launcher than the one already included.
Implementing the custom Widget launcher
Once the Widget is loaded and ready, the global `onsetWidget` instance exposes helpful methods that make using a custom launcher a breeze. In this tutorial, we will be focusing on the show, hide, and toggle functions.
show()
- shows the widget window.hide()
- hides the widget window.toggle()
- shows/hides based on the Widget's current state.
You can learn about all the available methods here.
There are various ways of integrating and using the custom launcher. It all depends on your setup. Here are two examples:
React
Here is a simple example of how to use the widget methods in React:
import React from 'react';
function Example() {
return (
<div>
<button onClick={() => window.onsetWidget?.show()}>
Show Widget
</button>
<button onClick={() => window.onsetWidget?.hide()}>
Hide Widget
</button>
<button onClick={() => window.onsetWidget?.toggle()}>
Toggle Widget
</button>
</div>
);
}
Plain JavaScript
The Widget doesn't rely on any frameworks or plugins from outside sources. Here is an example of how to build a custom launcher using HTML and simple JavaScript.
HTML
<button type="button" id="showWidget">Show Widget</button>
<button type="button" id="hideWidget">Hide Widget</button>
<button type="button" id="toggleWidget">Toggle Widget</button>
JavaScript
const showButton = document.getElementById('showWidget');
showButton.addEventListener('click', () => window.onsetWidget?.show(), false);
const hideButton = document.getElementById('hideWidget');
hideButton.addEventListener('click', () => window.onsetWidget?.show(), false);
const toggleButton = document.getElementById('toggleWidget');
toggleButton.addEventListener('click', () => window.onsetWidget?.toggle(), false);
The Widget's JavaScript API makes integration seamless. It provides a range of options, events, and methods that you can use to customize the Widget's appearance and behavior to fit your specific needs. The documentation provides detailed explanations of all available functionality, so you can quickly get up to speed with how to use the API effectively.